- Feb 21, 2013
- 3,202
- 535
- 175
I found that it cannot be simply set in panel properties but needs to be coded. Currently the Launcher is stuck in the middle of desktop.
I followed an example on YT which shows how you write code for mouseDown, mouseMove and mouseUp but VS doesn't like what I did and I suspect I need to move the code up to the very start, into the public MainForm() method as I marked in this image by a red rectangle.
When I selected mouseDown in the Events, VS entered the code for it smack at the bottom of the code for the Launcher and that put it alongside that public MainForm(), into the public partial class MainForm : Form
This is the code and I think I need to define mouseX and mouseY somehow... I thought that would be done by that 'int = mouseX = 0... line but evidently it is not.
So, how I make mouseX to exist in the current context? Same for mouseY...
It didn't put out the error underlines for the guy in the tutorial but of course he wrote the code in 'different place', in that method that I have at the very top of the code where I put the red rectangle.
Also his InitializeComponent(); is inside that public Form1() but in the Launcher code, that InitializeComponent is left 'as is'
I suppose I could move the new code into that top method but don't really believe that's what needs to be done.
I followed an example on YT which shows how you write code for mouseDown, mouseMove and mouseUp but VS doesn't like what I did and I suspect I need to move the code up to the very start, into the public MainForm() method as I marked in this image by a red rectangle.
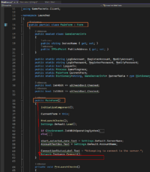
When I selected mouseDown in the Events, VS entered the code for it smack at the bottom of the code for the Launcher and that put it alongside that public MainForm(), into the public partial class MainForm : Form
This is the code and I think I need to define mouseX and mouseY somehow... I thought that would be done by that 'int = mouseX = 0... line but evidently it is not.

So, how I make mouseX to exist in the current context? Same for mouseY...

It didn't put out the error underlines for the guy in the tutorial but of course he wrote the code in 'different place', in that method that I have at the very top of the code where I put the red rectangle.
Also his InitializeComponent(); is inside that public Form1() but in the Launcher code, that InitializeComponent is left 'as is'

I suppose I could move the new code into that top method but don't really believe that's what needs to be done.
C#:
int = mouseX = 0, mouseY = 0;
bool mouseDown;
private void MainTab_MouseDown(object sender, MouseEventArgs e)
{
mouseDown = true;
}
private void MainTab_MouseMove(object sender, MouseEventArgs e)
{
if (mouseDown)
{
mouseX = MousePosition.X - 200;
mouseY = MousePosition.Y - 20;
this.SetDesktopLocation(mouseX, mouseY);
}
}
private void MainTab_MouseUp(object sender, MouseEventArgs e)
{
mouseDown = false;
}
}
}
Last edited: